Programming | Web Dev | Javascript
A beginner programmer’s experience with vanilla Js 30 challenge
My experience with Wes Bos’s vanilla javascript 30 challenge.
Hello everyone, first of all thanks a lot for coming here. I think it would be wise to inform you prior to the beginning of this article, this happens to be my first technical blog.
The reason I am writing this is because I think the best way to learn is by explaining and sharing it with others, helping others resolve their issues and by doing that, we a lot of times solve our own doubts and check our understanding of important concepts.
Since I have already mentioned in the title itself that I am a beginner programmer, by that I mean I am aware of programming basics and fundamentals but have not dived into much more important stuff just yet like data structures and algorithms.
I know that it may seem a bit vague as to what exactly a beginner level is, it may include or exclude a lot of important stuff but I think by mentioning a couple of things about myself you would better understand my position; neither I have a bachelors in computer science nor I did coding in my college but merely to satisfy my curiosity to program and make something useful, I intend to pursue coding.
I feel it is important to make my readers understand the place I come from so that they are able to gauge more precisely my ability to deal with the stuff I am writing about.
So here’s how it goes, we have 30 interesting projects which are expected to be finished in some 30 cool days, but the real question is “Is it even possible or it’s going to be way too easy that you may think that you will complete it in less than 30 days”.
Well no one can really answer that question without actually diving into it, but I before beginning the first project just made a simple commitment to myself that I will be consistent and will complete at-most one project per day.
Even if I have extra time in my hands rather than getting excited and taking on the next one I would simply chose to review what I had learnt, do more research about it or move on to something completely different.
So I broke these challenges down to single individual projects as they were given to me where not only I provide a brief description about the project in simplest terms but also I mentioned some of the key stuff we would be engaging with to get things done, furthermore, it is important to emphasize that the information provided will not divulge the solution to the reader. Instead, its purpose is to assist readers in gaining a clear understanding of the challenges they are about to encounter. Without further delay, let’s begin.
- Javascript Drum Kit

Welcome to the start of our exciting journey through the JavaScript 30 challenge. Our first undertaking, the JavaScript Drum Kit, serves as a formidable introduction, setting the bar high for the upcoming challenges. As a beginner-level programmer, you’ll quickly grasp that these tasks demand a solid understanding of JavaScript principles and creativity.
In the JavaScript Drum Kit, we dynamically integrate sound and animation with an onscreen keyboard. Leveraging both mouse clicks and keyboard inputs, we create a captivating project that allows users to produce drum-like sounds and visual effects. Throughout this challenge, you’ll explore event listeners, audio handling, and DOM manipulation, gaining valuable experience in implementing interactive features.
The drum kit’s implementation showcases the power of JavaScript in building interactive web elements. As we progress through the challenges, you’ll encounter a variety of such projects, each designed to enhance your proficiency and problem-solving skills. Let’s dive in and embrace the learning journey ahead!
2. CSS+JS Clock

The CSS + JS Clock challenge provides a fantastic opportunity to delve into the fundamentals of JavaScript. Through this project, we’ll reinforce essential concepts, such as selecting elements using querySelector(), utilizing functions effectively, and leveraging additional methods to simplify our coding tasks.
As we embark on this challenge, you’ll discover how to create a visually appealing clock interface using CSS styles combined with JavaScript’s dynamic capabilities. By employing querySelector(), we’ll target specific elements on the page, enabling us to manipulate their appearance and behavior programmatically.
Moreover, you’ll gain hands-on experience in building functions, a cornerstone of JavaScript development. These functions will be instrumental in updating the clock’s time dynamically and ensuring a smooth user experience.
By the end of the CSS + JS Clock challenge, you’ll have a deeper understanding of JavaScript’s role in crafting interactive user interfaces and the importance of organizing code into reusable functions.
3. Playing with CSS variables and JS

The Playing with CSS Variables and JS challenge proved to be an eye-opening experience for me. Before undertaking this exercise, I was unaware that CSS allows us to define variables and that we can leverage JavaScript to dynamically alter their values during runtime. This insightful exercise focused on CSS variables and their remarkable capabilities.
As I delved into this challenge, I discovered the power of CSS variables, also known as custom properties. They provide a way to store and reuse values throughout our stylesheets, streamlining the process of maintaining and modifying our designs. The dynamic nature of these variables, coupled with JavaScript’s intervention, allows us to create more interactive and adaptable user interfaces.
Through this exercise, I honed my skills in manipulating CSS variables using JavaScript. I learned how to access and modify these variables programmatically, giving me a newfound appreciation for the synergy between CSS and JavaScript in web development.
4. Array Cardio 1

The Array Cardio 1 challenge, led by our witty guide and teacher Wes, sets the tone for an engaging learning experience. From the outset, Wes emphasizes the importance of familiarizing ourselves with fundamental JavaScript methods frequently used in functional programming. This exercise serves as an excellent platform to focus on essential array manipulation methods.
Throughout this challenge, we delve into various array methods, discovering their diverse applications and usefulness in data processing and manipulation. By mastering these methods, we gain valuable skills in working with arrays efficiently and effectively.
The array methods covered in this exercise equip us with powerful tools for filtering, mapping, sorting, and reducing arrays. Understanding their proper usage is crucial for creating clean and concise code, a hallmark of functional programming.
As we progress through this enlightening exercise, we uncover the elegance of functional programming paradigms and the advantages they bring to our JavaScript projects. Armed with these newfound skills, we are better prepared to tackle complex data transformations and streamline our codebase.
5. Flex panels image gallery

The Flex Panels Image Gallery challenge proved to be an immersive exploration of key CSS concepts, including flexbox, transitions, and animations. As I delved into this exercise, I realized the significance of these CSS techniques in creating dynamic and visually appealing web layouts.
My initial encounter with flexbox was an enlightening experience, as it opened up a new realm of possibilities in web design and layout structuring. Understanding the fundamentals of flexbox was paramount to successfully implementing the flexible panels in this image gallery project.
Moreover, incorporating transitions and animations further elevated the user experience, as the panels smoothly expanded and revealed their content. The careful synchronization of JavaScript with CSS transitions enabled seamless interactivity, captivating users as they interacted with the image gallery.
Although it required dedicated time and effort, my journey into CSS for this challenge was both rewarding and enlightening. The knowledge gained in mastering flexbox, transitions, and animations has significantly enhanced my skills as a web developer.
The Flex Panels Image Gallery project stands as a testament to the power of CSS in transforming static layouts into engaging and interactive experiences. It serves as a stepping stone toward more sophisticated projects, where I will continue to leverage these CSS techniques to craft modern and visually stunning web applications.
6. Ajax type ahead

The Ajax Type Ahead challenge leads us to create an impressive type ahead feature, allowing users to explore a comprehensive list of cities and states, complete with their corresponding populations. As we type, the application dynamically filters and displays matching results, offering a seamless search experience.
To build this functionality, we leverage the power of asynchronous programming and AJAX. The data for cities and states resides in an external JSON file, and we utilize the fetch API to request and fetch this data asynchronously. The fetch API returns a promise that resolves into a response containing the required data.
Handling the promise effectively enables us to retrieve and process the data seamlessly, ensuring a smooth user experience. We employ JavaScript to manipulate the fetched data, dynamically updating the display as users input their search queries.
By mastering the Ajax Type Ahead challenge, we delve into the world of asynchronous programming and explore the fetch API’s capabilities in handling external data. This exercise equips us with valuable skills for managing data in real-world applications, where we often interact with APIs to provide dynamic and responsive content.
7. Array Cardio 2
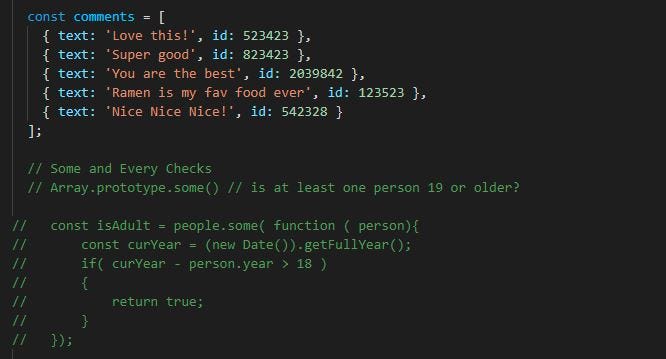
Array Cardio 2 serves as a seamless extension of the skills developed in Array Cardio 1, as indicated by its name. In this advanced challenge, we dive even deeper into essential JavaScript methods, enriching our problem-solving toolkit for future endeavors.
Building upon the foundation laid in the previous challenge, Array Cardio 2 introduces us to a set of additional JavaScript methods that are invaluable in handling complex data manipulations. These methods unlock new possibilities for data filtering, mapping, and reducing, empowering us to write more efficient and concise code.
Throughout this exercise, we encounter real-world scenarios where these methods shine, solving problems with elegance and precision. By mastering these array methods, we enhance our proficiency in managing data structures and preparing them for various data transformations.
The knowledge gained from Array Cardio 2 is a significant asset as we progress through the JavaScript 30 series and beyond. Armed with a comprehensive understanding of these essential methods, we become better-equipped developers, capable of tackling a wide array of programming challenges.
8. Fun with HTML 5 canvas
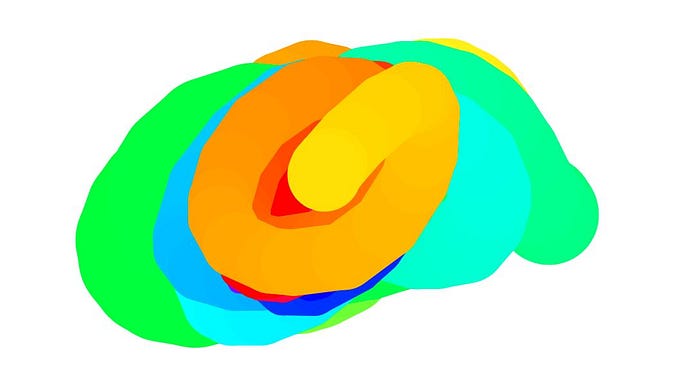
Fun with HTML5 Canvas presents a delightful surprise as we explore the powerful canvas element in HTML, opening up a world of possibilities for drawing graphics using JavaScript. This versatile feature not only enables us to create stunning visuals but also serves as a foundation for animation, game graphics, data visualization, photo manipulation, and even real-time video processing.
Discovering the capabilities of the canvas element was a remarkable moment for me, as it introduced a whole new dimension to what can be achieved using JavaScript. The canvas element empowers us to dynamically generate and manipulate graphics directly on the web page, providing an interactive and engaging experience for users.
This challenge encourages us to dive into creative graphics work, unleashing our imagination and experimenting with various techniques to bring our ideas to life. Whether it’s designing animations, interactive games, or data visualizations, the canvas element allows us to craft unique and visually appealing projects.
As we embark on this exciting journey with HTML5 Canvas, we will witness the potential of combining JavaScript’s dynamic capabilities with graphical creativity. The knowledge gained from this challenge will undoubtedly inspire us to explore further and push the boundaries of what we can achieve with JavaScript and HTML.
9. Dev tool tricks

As we progress through the challenges and reach exercise 9, we undoubtedly become more adept at solving problems using JavaScript. However, to further elevate our problem-solving process and gain deeper insights into core issues, exploring some cool tools and tricks within developer tools becomes essential.
The Dev Tool Tricks challenge introduces us to a set of powerful tools and techniques that can significantly enhance our productivity and debugging capabilities.
By leveraging these tools, we gain the ability to inspect and debug our code with precision, understanding how each line of JavaScript affects the application’s behavior. This knowledge empowers us to optimize our code for performance and efficiency, delivering a smoother and more responsive user experience.
Moreover, exploring these dev tool tricks widens our horizons, granting us a deeper understanding of web applications’ inner workings. We can analyze network requests, examine performance bottlenecks, and visualize data flow, enabling us to make informed decisions and tackle complex issues effectively.
10. Hold Shift to Check Multiple CheckBoxes
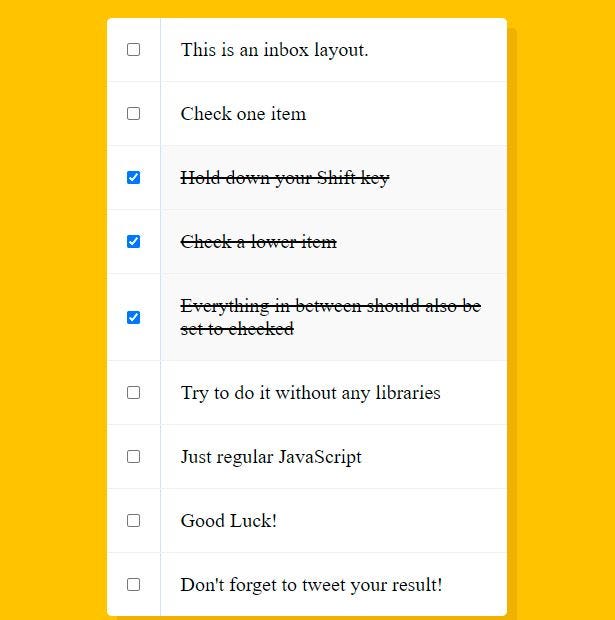
The Hold Shift to Check Multiple Checkboxes challenge takes inspiration from a common yet crucial functionality found in Gmail. Our task is to implement a feature that enables users to select multiple checkboxes by holding the Shift button and clicking on the first and second elements.
This seemingly tricky project, as Wes describes in the beginning, encourages viewers to engage their problem-solving skills independently before exploring his approach. It serves as an excellent opportunity to put the knowledge acquired thus far to the test, demonstrating our ability to apply JavaScript concepts creatively.
Implementing this functionality requires a thoughtful approach, involving event listeners, array manipulation, and conditional logic. As we tackle this challenge, we consolidate our understanding of handling user interactions and modifying the DOM dynamically.
11. Custom HTML 5 video player

The Custom HTML5 Video Player challenge stands out as a significant milestone in the initial 11 exercises, presenting a more comprehensive project with a considerable number of elements to consider. While initially feeling a bit heavy, this project proved to be a valuable learning experience, teaching us essential strategies to tackle complex tasks effectively.
As we worked through this challenge, we learned the importance of breaking down larger problems into smaller, manageable tasks. By creating separate functions for each task, we embraced a more organized and structured coding approach. This enabled us to maintain better control over the project’s complexity, making it easier to debug and optimize our code.
Furthermore, leveraging objects and their properties became a game-changer in reducing code redundancy and enhancing readability. By encapsulating related functionalities within objects, we improved code maintainability and achieved a more elegant implementation.
The process of building a custom video player showcased the versatility of JavaScript in manipulating HTML5 video elements. We learned how to control video playback, adjust volume and playback rate, add custom controls, and respond to user interactions.
This exercise opened our eyes to the possibilities of creating interactive and engaging media experiences on the web. It served as a foundation for future projects involving multimedia integration and user-centric interfaces.
As we continue our JavaScript journey, we carry forward these valuable lessons in problem-solving, code organization, and object-oriented programming. With each new challenge, we grow as developers and unlock the potential to create innovative and user-friendly web applications.
12. Key Sequence Detection

In the Key Sequence Detection challenge, we embark on an intriguing project that involves detecting a specific sequence of key presses to trigger a desired output. The secret code, known only to us, unlocks a hidden functionality when entered correctly.
To achieve this functionality, we delve into key event handling in JavaScript, allowing us to capture the key codes generated by user input. By monitoring the sequence of key presses, we can compare them to the secret code and activate the desired action once the correct sequence is detected.
This project showcases the power of JavaScript in capturing and interpreting user interactions, providing an interactive and engaging experience. The concept of key sequence detection is commonly used in various applications, adding an extra layer of security or facilitating hidden features.
As we work through this challenge, we sharpen our skills in handling events, manipulating key codes, and implementing conditional logic. The knowledge gained from this exercise reinforces our understanding of how JavaScript can respond to user input dynamically.
13. Slide in on Scroll

The Slide in on Scroll challenge presents a remarkably elegant behavior that adds a touch of magic to our web pages. As we scroll down the page, images gracefully slide into view under specific conditions, creating a visually captivating effect.
Implementing this smooth slide-in effect demands a creative approach and a keen understanding of JavaScript and CSS. We explore event listeners and the scroll event, closely monitoring the user’s scrolling behavior to trigger the animation when the images enter the viewport.
The result is a delightful user experience, where images seamlessly come into focus as users navigate through the content. This captivating behavior enhances the visual appeal of our web pages, making them more engaging and memorable.
As we work on this challenge, we gain valuable insights into combining JavaScript and CSS to create dynamic and interactive web elements. We discover how animations can bring a sense of life and personality to otherwise static images, elevating the overall user experience.
The Slide in on Scroll challenge allows us to delve into the art of storytelling through web design, strategically presenting images at just the right moment to evoke emotions and intrigue the audience.
14. Objects and Arrays ( Reference vs Copy )

The Objects and Arrays (Reference vs. Copy) challenge sheds light on a common area of confusion among programmers: the distinction between making a reference and making a copy, particularly when dealing with arrays. Thanks to Wes’s expert guidance and simple exercises, we gain clarity on this topic and extend our gratitude to him for his invaluable teachings.
Understanding whether we are creating a reference or a copy is crucial, as it affects how we manipulate data and ensures that our code behaves as expected. Through hands-on exercises, we delve into the intricacies of working with objects and arrays, gaining insights into their behavior when assigned or passed as variables.
By the end of this challenge, we are equipped with the knowledge to confidently manage objects and arrays, applying the correct approach for various scenarios. The distinction between references and copies opens up new possibilities in our coding arsenal, enabling us to create efficient and reliable solutions.
15. Local Storage and Event Delegation

The Local Storage and Event Delegation challenge demystifies the mystery behind how browser-based games store data, such as the highest scores. The solution lies in utilizing the local storage read-only property of the window interface, a powerful feature that enables us to persist data locally in the user’s browser.
Through this exercise, we not only learn how to store and retrieve data using local storage but also explore important concepts like converting objects to JSON string equivalents for storage and vice versa. We also discover efficient ways to manage data, such as pushing items into arrays, providing a foundation for more sophisticated data handling in our projects.
Event delegation, another crucial aspect covered in this challenge, allows us to handle events more efficiently by attaching a single event listener to a parent element instead of individual elements. This technique proves invaluable in scenarios where we have dynamically generated elements or large lists.
By the end of this project, we gain invaluable insights into how data is managed in local storage and understand the power it brings to creating feature-rich applications. The ability to store data locally opens up endless possibilities for creating personalized user experiences and implementing game-saving features.
16. CSS text shadow mouse move effect

The CSS Text Shadow Mouse Move Effect challenge introduces us to the magic of cool effects that can be triggered by user interactions, such as hovering or clicking on items. In this project, we harness the power of JavaScript to render dynamic styling and create captivating text shadow mouse effects.
As we move the mouse over the text, we witness the shadows elegantly transform, adding a touch of interactivity and creativity to our web elements. This engaging behavior enhances the user experience and leaves a lasting impression on visitors.
By combining JavaScript and CSS, we create an enchanting visual display, showcasing the versatility of web development tools. This exercise not only allows us to experiment with exciting mouse effects but also encourages us to explore innovative ways of engaging users through interactive design.
The CSS Text Shadow Mouse Move Effect project offers a valuable opportunity to improve our skills in JavaScript event handling and DOM manipulation. Understanding how to respond to mouse movements empowers us to create more immersive and user-friendly interfaces.
Through this challenge, we embrace the artistry of web design, transforming static elements into living, breathing components that respond to user interactions. By mastering this technique, we add a touch of elegance to our web applications and keep users enchanted by the magic of the web.
17. Sorting Band Names without articles

The Sorting Band Names Without Articles challenge provided an excellent opportunity to explore the array.sort() method in JavaScript and its application in sorting an array of band names while ignoring articles. This neat exercise delves into an essential JavaScript method, equipping us with valuable skills in data manipulation and sorting.
The task of sorting band names without articles, such as ‘The,’ ‘A,’ or ‘An,’ may seem simple, but it requires a thoughtful approach to ensure accurate results. By leveraging array.sort() and understanding its underlying sorting algorithm, we can efficiently rearrange the band names to create a more organized and user-friendly list.
This exercise deepens our appreciation for the power and versatility of JavaScript’s built-in methods, providing a glimpse into the vast capabilities of this popular programming language.
The Sorting Band Names Without Articles challenge encourages us to think critically and creatively when solving real-world coding problems. As we gain familiarity with array.sort(), we enhance our problem-solving skills and become more proficient in handling data and arrays in JavaScript.
18. Tally string time with reduce

The Tally String Time with Reduce challenge offers a simple and enjoyable exercise that not only satisfies our curiosity but also highlights the significance of the reduce method in JavaScript. Embarking on this project reveals the incredible power and versatility that reduce brings to our coding toolkit.
Through this exercise, we explore the magic of reduce, learning how it efficiently accumulates values and simplifies complex data manipulations. Tallying string time may seem like a small task, but it uncovers the vast potential of this essential JavaScript method.
As we work on this challenge, we gain a deeper appreciation for the functional programming paradigm, where reduce plays a pivotal role in streamlining data processing and transforming arrays.
By leveraging reduce, we can elegantly calculate the total duration of time represented in strings, such as ‘2:30’ (2 minutes and 30 seconds) and ‘1:45’ (1 minute and 45 seconds), to arrive at a comprehensive tally.
The Tally String Time with Reduce challenge urges us to embrace the power of higher-order functions in JavaScript and encourages us to seek creative solutions to real-world problems. It sparks our curiosity and pushes us to explore more possibilities with this versatile language.
19. Unreal Webcam Fun

The Unreal Webcam Fun challenge brings a delightful surprise as we venture into building a photobooth straight out of our imaginations. Who would have thought we’d have the opportunity to create something so fun and interactive?
Through this project, we immerse ourselves in a world where data flows seamlessly from the webcam to the video element in HTML, and then onto the canvas element, allowing us to explore creative possibilities and manipulate real-time images.
The photobooth functionality captures the essence of playful experimentation, as we can take photos and even download them to preserve our moments of creativity.
The process of building this photobooth reinforces our understanding of working with multimedia elements in HTML and CSS, as well as the powerful capabilities of JavaScript in managing user interactions and dynamic data manipulation.
As we play with data streams and canvas manipulation, we develop a deeper appreciation for the potential of web development to provide engaging and immersive experiences.
20. Native Speech Recognition

The Native Speech Recognition challenge introduces us to the fascinating world of speech-to-text conversion, made possible by the powerful Web Speech API. This innovative API empowers us to integrate voice data seamlessly into web applications, transforming the way users interact with our creations.
Comprising two essential components, the Web Speech API consists of SpeechSynthesis, which enables text-to-speech functionality, and SpeechRecognition, our focus in this project. SpeechRecognition offers asynchronous speech recognition capabilities, enabling us to convert voice input into actionable text data.
As we delve into this challenge, we explore the endless possibilities that voice recognition brings to web development. By tapping into the SpeechRecognition capabilities, we can provide users with hands-free interaction, making our applications more accessible and user-friendly.
The Native Speech Recognition project enhances our understanding of asynchronous JavaScript programming, as we handle voice input asynchronously and respond to it in real-time.
Embracing the Web Speech API is a testament to the ever-evolving nature of web development, where we constantly strive to enhance user experiences by leveraging cutting-edge technologies.
By completing this challenge, we gain valuable insights into the potential of voice-driven interfaces, an increasingly prevalent trend in modern web development. This knowledge opens doors to explore voice-controlled applications, virtual assistants, and beyond.
21. Geolocation based speedometer and compass

The Geolocation Based Speedometer and Compass challenge presents an exciting project that harnesses the power of geolocation to create a dynamic speedometer and compass display. By tapping into the user’s device location, we gain access to latitude and longitude data, among other properties, to create an engaging and interactive user experience.
Through this project, we delve into the realm of geolocation, where we leverage JavaScript’s Geolocation API to access the user’s current location in real-time. This opens up a wealth of possibilities for developing location-based web applications and features.
As we extract and utilize the geolocation data, we craft a captivating speedometer display that visually represents the user’s speed and direction. This adds a touch of practicality and fun to our web application, making it stand out from traditional static interfaces.
The combination of JavaScript for data manipulation and CSS for styling allows us to create a visually appealing and responsive user interface. By skillfully blending these technologies, we gain the ability to present real-world data in an engaging and meaningful way.
Beyond the technical aspects, this challenge offers valuable insights into geospatial web development, paving the way for more complex location-based applications. It demonstrates the potential of using geolocation data to deliver personalized and location-aware user experiences.
22. Follow Along Links

The Follow Along Links challenge satisfies our curiosity about those captivating highlighted links that come to life when the cursor hovers over them on a web page. Through this project, we have the opportunity to bring this engaging effect to our own creations, leaving a sense of accomplishment and excitement.
Implementing the Follow Along Links effect involves skillfully blending CSS and JavaScript to create the illusion of a background expanding around the links as the cursor hovers over them. This captivating animation adds a touch of interactivity to our user interfaces, elevating the overall user experience.
As we master the art of follow-along links, we gain valuable insights into working with CSS animations and event handling in JavaScript. This knowledge enhances our ability to craft delightful user experiences, ensuring our web applications stand out from the crowd.
23. Speech Synthesis

The Speech Synthesis challenge undoubtedly stands out as one of the coolest projects I have ever undertaken. Bringing text-to-speech capabilities to life, this project introduces us to two crucial components that make it all possible.
First and foremost, we encounter the Speech Synthesis Utterance interface, an essential part of the Web Speech API. This powerful interface represents a speech request, enabling us to convert text into speech with ease. The Speech Synthesis Utterance allows us to customize and control the speech synthesis process, adding a personal touch to our projects.
Secondly, we explore the speech synthesis variable, a key player that resides in the browser. This variable serves as the core engine behind text-to-speech functionality, seamlessly transforming text into audible speech.
The combination of these components opens up a world of possibilities for making our web applications more inclusive and accessible. By providing text-to-speech capabilities, we cater to a wider audience, including individuals with visual impairments or those seeking a more interactive and engaging user experience.
The Speech Synthesis challenge delves into the realm of voice interfaces, a rapidly evolving area of web development that holds great promise for the future. As we master text-to-speech capabilities, we gain invaluable insights into enhancing user interactions and creating more engaging applications.
24. Sticky Nav

The Sticky Nav challenge explores the art of creating a seamless scrolling experience on a web page by applying captivating effects. Through this exercise, we uncover the magic of CSS properties working in harmony with JavaScript to achieve a sticky navigation bar that gracefully adapts as users scroll through the content.
The concept of a sticky navigation bar adds a touch of elegance to web design, providing users with quick access to essential menu options, no matter where they are on the page. This seamless experience enhances user navigation and engagement, making our web applications more user-friendly.
By combining CSS properties and JavaScript, we bring this vision to life, ensuring that our navigation bar stays fixed at the top of the viewport as users scroll down the page. This dynamic behavior is achieved through careful event handling and CSS manipulation, creating a smooth and visually appealing transition.
The Sticky Nav challenge not only enhances our proficiency in CSS and JavaScript but also instills a deeper understanding of user experience design. As we refine the scrolling effects and animations, we gain insights into crafting user-centric interfaces that keep users immersed in our content.
25. Event Capture, Propagation, Bubbling and Once

The Event Capture, Propagation, Bubbling, and Once challenge delves into fundamental concepts associated with JavaScript event handling. Understanding these concepts is crucial for building robust and sophisticated web applications that respond to user interactions seamlessly.
Through this exercise, we explore four key concepts:
- Event Capture: The process of capturing events as they travel down the DOM tree, from the top-level ancestor to the target element. Understanding event capture allows us to intercept events early in the propagation process, making it ideal for certain use cases.
- Propagation: The journey of an event from the target element to its ancestors, bubbling up through the DOM tree. Properly managing event propagation is essential to control how events are handled as they traverse the DOM.
- Bubbling: The phenomenon where events bubble up through the DOM tree from the target element to its ancestors. Harnessing event bubbling enables us to handle events more efficiently, reducing the need for attaching multiple event listeners.
- Once: A feature that allows an event listener to execute only once, effectively removing the listener after the first execution. The ‘once’ option optimizes performance and prevents unnecessary event handling.
By mastering these concepts, we unlock the power to create responsive and interactive web applications. With a solid understanding of event handling, we can build complex user interfaces with ease, improving the overall user experience.
The Event Capture, Propagation, Bubbling, and Once challenge opens the door to more advanced event handling techniques and encourages us to explore innovative ways of managing user interactions.
26. Follow Along Dropdown

The Follow Along Dropdown challenge presents an exciting extension of our previous project, Follow Along Links. Building upon the cool functionality of the previous exercise, this project takes interactivity to the next level by creating an elegant dropdown effect that responds to user actions with finesse.
The heart of this challenge lies in CSS manipulation, as we adjust essential properties such as transition time and opacity to orchestrate the smooth follow-along background movement. As users hover over the links, the dropdown gracefully appears, enhancing the visual appeal and user experience of our web application.
The Follow Along Dropdown is a showcase of how combining CSS animations and JavaScript event handling can lead to captivating user interactions. This project empowers us to transform a simple navigation element into a dynamic and engaging component that keeps users intrigued.
Understanding the intricate dance of CSS transitions and event listeners enables us to craft elegant and seamless dropdowns that add a touch of sophistication to our web interfaces.
By mastering the art of follow-along effects, we add a new layer of creativity to our web development repertoire. The ability to create fluid and intuitive dropdowns opens up exciting possibilities for improving navigation and content discovery on our websites.
27. Click and Drag to Scroll

The Click and Drag to Scroll challenge introduces us to an engaging and intuitive functionality that we often encounter on modern websites. You might have experienced this feature, where items can be clicked and dragged to scroll horizontally, revealing more content in a dynamic and interactive manner.
This seemingly simple yet powerful functionality brings delight to user interactions, making the browsing experience more immersive and enjoyable. As we dive into coding this feature, we discover the art of handling significant mouse events that enable seamless click and drag interactions.
Working with mouse events like ‘mousedown,’ ‘mousemove,’ and ‘mouseup,’ we unleash the potential to create fluid and responsive scrolling behaviors. The careful coordination of these events facilitates smooth and intuitive scrolling, leaving users with a sense of satisfaction as they interact with our web application.
The Click and Drag to Scroll challenge showcases how combining CSS styling and JavaScript event handling transforms a basic container into a captivating and user-friendly scrolling component.
28. Video Speed Controller UI

The Video Speed Controller UI challenge delves into a critical aspect of video player interfaces — the playback rate control. This essential feature allows users, like myself and many others, to watch videos at varying speeds, customizing the viewing experience to match our preferences. The significance of this element in a video player cannot be overstated, as it empowers us to control the playback pace and engage with content in a more personalized manner.
By crafting a sleek and functional video speed controller, we enhance the usability and interactivity of our video player. The ability to seamlessly adjust the playback rate adds a layer of convenience and accessibility, ensuring that users can consume content at their preferred speed.
As we complete this task, we gain a profound sense of achievement, knowing that we have contributed to creating a more user-friendly and intuitive video playback experience.
The Video Speed Controller UI challenge also hones our skills in JavaScript event handling and DOM manipulation. By efficiently capturing user input and synchronizing it with the video playback, we showcase the power of technology in enhancing multimedia interactions.
Building the Video Speed Controller UI offers a glimpse into the intricacies of video player design and development. As we perfect the interface for playback rate control, we become more adept at creating feature-rich and user-centric applications.
29. Countdown Clock

The Countdown Clock challenge strikes close to home, as it addresses a practical aspect of our daily lives. We often find ourselves engaged in tasks with time constraints, and uncertainty about the remaining time can lead to unnecessary anxiety and stress. This project offers a powerful solution — an elegant countdown timer that provides precise estimates of the time left, empowering us with control and certainty.
As we embark on this challenge, we venture into the realm of time manipulation and JavaScript date handling. By skillfully coding the countdown timer, we enhance our understanding of date and time functions, enabling us to create dynamic and functional user interfaces.
The Countdown Clock project brings practicality and convenience to our lives, whether we are working on a project with a deadline or simply seeking a mindful approach to time management. By incorporating this feature into our applications, we ensure smoother task execution and a heightened sense of focus and productivity.
Beyond the technical aspects, this project carries significant real-life relevance, reflecting the value of user-centric design in web development. Empowering users with accurate and intuitive countdowns transforms our applications into valuable tools that enhance efficiency and effectiveness.
As we complete this cool second last project, we relish the satisfaction of crafting a feature that adds tangible value to our lives. The ability to control and manage time with certainty is a testament to the power of technology in enriching our everyday experiences.
30. WHACK A MOLE GAME
The Whack A Mole Game marks the culmination of our learning journey, where we put together all the tools and concepts we have acquired so far. Throughout the challenges, we have mastered selecting elements using JavaScript, declaring variables, working with APIs, writing various functions, dealing with higher-order functions, utilizing flag variables, and managing event listeners to call functions.
As we embark on building this exciting game, we encounter a vital programming technique — recursion. This powerful concept allows us to create self-referential functions that repeat a specific task until a certain condition is met. In the context of Whack A Mole, recursion becomes a crucial aspect of the game’s mechanics, contributing to its dynamic and engaging gameplay.
The process of constructing the Whack A Mole Game involves meticulous planning and organization. We set up functions tailored to different tasks and functionalities, skillfully utilizing global and local variables to manage game states and player interactions.
Designing the game interface and logic showcases the art of combining creativity and technical expertise. We craft an immersive and enjoyable user experience, where players can test their reflexes and aim to whack the elusive moles as they pop up.
The Whack A Mole Game epitomizes the power of programming to create interactive and entertaining applications. As we celebrate completing this final challenge, we recognize the transformation we have undergone as web developers, equipped with the skills to build captivating projects from scratch.
Constructing a game as the final project in this course proved to be an excellent decision. Not only did it boost my confidence in development skills, but it also motivated me to tackle more complex projects that I had previously been hesitant to approach. Through practice and hands-on experience, I gained the courage to venture into sophisticated endeavors.
As Wes aptly pointed out, the completion of 30 vanilla JavaScript projects is merely the starting point. There is a vast realm of knowledge waiting to be conquered. This journey brought me closer to comprehending his tagline, prominently displayed on his homepage:
“There is no formation without repetition”.
Indeed, repetition and consistent effort are fundamental to mastery.
If you’ve reached this point in the article, I genuinely appreciate your time and attention. For those embarking on their programming journey as beginners, I encourage you to take up the challenge without hesitation. Remember, the key is to “don’t think, Do it!” Thank you for joining me on this exploration.